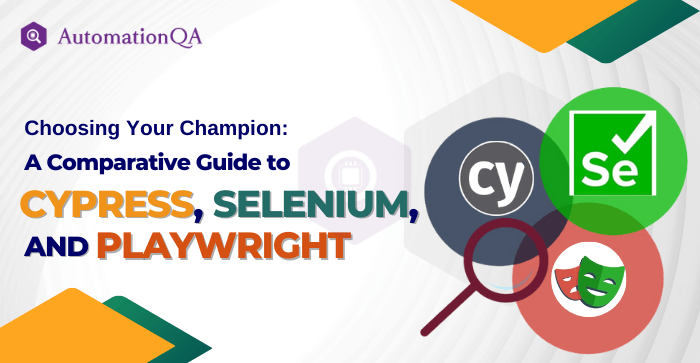
When choosing a cross-browser testing tool, it’s essential to consider your specific needs. Selenium has been the default choice for more than a decade. However, newer alternatives, such as Cypress and Playwright, are garnering attention and adoption. These tools provide special capabilities and advantages to meet various testing needs. Even a recent survey by the State of JS also corroborates this statement.
Automation tools are critical to the success of any product. Delivering a top-notch application requires managing difficult situations, which a flawless tool can accomplish. But deciding between Selenium, Cypress, and Playwright can be overwhelming even for a seasoned tester. Therefore, this article will help you make an informed decision by telling you about the strengths and weaknesses of each automation testing tool. By the end of this blog, you will be able to select the best tool for your project.
Selenium
It is a veteran in the testing industry and is well-known for its versatility. Additionally, it offers comprehensive programming language support and compatibility across various browsers. Its components, such as WebDriver, IDE, and Grid, make it unique. These components can address varying degrees of intricacy in online testing. Several renowned brands, including Microsoft, Amazon, and IBM, trust Selenium to test their websites. But why? It’s because Selenium boasts a mature and highly compatible ecosystem. This ecosystem guarantees reliability for Selenium automation companies by ensuring seamless performance across diverse browsers and platforms. This is made possible by Selenium’s large community of users, who are helpful and supportive in making testing more accessible.
Pros of Selenium
1. Wide Browser Support: Selenium offers versatility across several operating systems, including Linux.
2. Language Support: Selenium provides bindings for Java, Python, C#, JavaScript, etc.. Thus, it caters to the needs of developers using different programming languages.
3. Large Community: With a vast and active community, Selenium offers ample documentation, resources, and user-generated content for troubleshooting and learning.
4. Robust Ecosystem: The Selenium testing services ecosystem includes tools like Selenium Grid for parallel testing, WebDriver for automation, and IDE for record-and-playback functionalities.
Cons of Selenium
1. Complex Setup: Initial setup, especially configuring drivers for various browsers, can be challenging for newcomers.
2. Steep Learning Curve: Due to its flexibility, Selenium has a steep learning curve, especially for those who are not familiar with test automation.
3. Lack of Native API Interaction: Selenium doesn’t have native support to interact with browser-executed APIs.
Cypress
It is an end-to-end testing framework tailored for modern web apps. One notable feature of Cypress is that it runs directly in the browser. This unique feature provides Cypress automation services with instant feedback during the creation and execution of the test. For instance, Cypress’s real-time reloading feature allows developers to see changes in their code immediately without the need to refresh the page manually. However, this is not the only reason why Cypress has gained popularity. In addition to its unique features, Cypress is well-known for its developer-friendly nature. Its intuitive interface makes testing efficient and easy, even for a beginner.
Moreover, Cypress simplifies testing by automatically handling waits for elements, making your test scripts more reliable and straightforward. Moreover, its built-in debugger empowers you with a step-by-step inspection of test runs, significantly enhancing your debugging and development efficiency.
Pros of Cypress
1. User-Friendly: Cypress automation tool offers a developer-friendly interface, making test writing and execution straightforward.
2. Real-Time Reloading: Its real-time reloading feature enhances test development and debugging efficiency.
3. Automatic Waiting: Cypress automatically waits for components, eliminating the need for explicit wait instructions in tests.
4. Time-Travel Debugging: Its time-travel debugging allows step-by-step inspection and debugging of test execution.
5. Fast Execution: Cypress is optimized for speed, resulting in faster test execution times than other tools.
Cons of Cypress
1. Limited Cross-Browser Support: Cypress has restricted support for browsers other than Chrome, which may hamper cross-browser testing.
2. No Mobile App Testing: Cypress is a testing tool designed primarily for web app testing; it lacks native capability for testing mobile applications.
3. Learning Curve for Advanced Scenarios: Cypress is easy for a beginner to use for basic tasks. However, a more in-depth knowledge of its architecture will be necessary for sophisticated testing situations.
Playwright
It is an open-source automation tool developed by Microsoft. It offers robust automation features like recording tests and taking screenshots during execution. Playwright’s concise API makes it a preferred choice for browser automation tasks. Its multi-browser support is beneficial for maintaining quality and compatibility across platforms. This makes the Playwright testing tool valuable for web testing, especially when cross-browser compatibility is crucial.
Pros of Playwright
1. Cross-Browser Support: Playwright allows extensive cross-browser testing by supporting several browsers, including Chromium, Firefox, and WebKit.
2. Unified API: Playwright’s unified API for web, mobile, and desktop apps makes it versatile for testing scenarios.
3. Handling Complex Scenarios: It excels at automating complex tasks like iframes, shadow DOM, and challenging elements, making it ideal for modern web apps.
4. Parallel Test Execution: Playwright natively supports parallel test execution, thereby simplifying the process compared to custom frameworks in Selenium.
5. Language Support: Playwright provides bindings for Java, Python, C#, JavaScript, etc., enabling developers working with various computer languages to access it.
Cons of Playwright
1. Learning Curve: For individuals accustomed to conventional automation tools, the learning curve for Playwright automation testing is quite high.
2. Smaller Community: Playwright has a smaller community than Selenium, which can result in fewer resources and content created by the community being available.
3. Compatibility Challenges: Playwright is updated often, leading to users reporting compatibility problems with some websites and programs that need to be adjusted in current codebases.
Cypress Vs. Selenium Vs. Playwright
Here’s a comparison of Cypress, Selenium, and Playwright based on various features:
Features | Cypress | Selenium | Playwright |
---|---|---|---|
Language Support | JavaScript | Multi-Language Support (Java, C#, Python, etc.) | Multi-Language Support (Java, C#, Python, etc.) |
Browser Support | Chrome, Firefox, Edge, Electron | Chrome, Firefox, Safari, Edge, Opera | Chrome & Edge with Chromium, Safari with Webkit, Firefox |
Architecture | Single-Threaded, Built-In Queuing | Multi-Threaded, Driver-Based | Single-Threaded, Built-In Queuing |
Execution Speed | Comparatively Fast | Comparatively Slow | Comparatively Fast |
DOM Manipulation | Powerful, Built-In Commands | Limited, Relies On Webdriver APIs | Powerful, Built-In Commands |
Cross-Browser Testing | Built-in support for cross-browser testing | Requires browser configuration | It also has a built-in support for cross-browser testing |
Headless Testing | Built-In Support | Requires Headless Mode Configuration | Built-In-Support |
Test Runner | Built-In | Requires External Test Runners | Built-In |
Debugging Support | Easy Debugging With Chrome Devtools | Limited Debugging Capabilities | Easy Debugging With Devtools |
Parallel Execution | Supports Using CI/CD Tool | Requires Additional Setup | Built-In Support |
Code Snippets
Here is the code snippet from different testing tools for login tests:
Cypress
```javascript
describe("Login Test", () => {
it("should log in successfully", () => {
cy.visit("https://the-internet.herokuapp.com/login");
cy.get("#username").type("tomsmith");
cy.get("#password").type("SuperSecretPassword!").type("{enter}");
cy.get("#flash").should("contain", "You logged into a secure area");
});
});
```
Selenium
```javascript
const { Builder, By, Key } = require('selenium-webdriver');
const assert = require('assert');
async function loginTest() {
const driver = new Builder().forBrowser('chrome').build();
try {
await driver.get('https://the-internet.herokuapp.com/login');
await driver.findElement(By.id('username')).sendKeys('tomsmith');
awaitdriver.findElement(By.id('password')).sendKeys('SuperSecretPassword!', Key.RETURN);
const successMessage = await driver.findElement(By.id('flash')).getText();
assert.ok(successMessage.includes('You logged into a secure area'), 'Login failed');
} finally {
await driver.quit();
}
}
loginTest();
```
Playwright
```javascript
const { chromium } = require('playwright');
const assert = require('assert');
async function loginTest() {
const browser = await chromium.launch({ headless: false });
const page = await browser.newPage();
await page.goto('https://the-internet.herokuapp.com/login');
await page.fill('#username', 'tomsmith');
await page.fill('#password', 'SuperSecretPassword!');
await page.press('#password', 'Enter');
await page.waitForSelector('#flash');
const successMessage = await page.innerText('#flash');
assert.ok(successMessage.includes('You logged into a secure area'), 'Login failed');
await browser.close();
}
loginTest();
```
Execution Time Comparison
The execution times for the scripts in interactive browser mode are as follows:
- Cypress: 5 seconds
- Selenium: 9.547 seconds
- Playwright: 4.657 seconds
Outcome
When deciding between Selenium, Playwright, and Cypress, consider your testing requirements and the characteristics of your application. The Playwright testing tool is versatile and has multi-browser support, making it an excellent choice for comprehensive cross-browser testing across various domains. Cypress shines in front-end testing. It offers excellent documentation and an interactive debugging experience.
At the same time, Selenium offers multi-language support, powerful built-in commands for cross-browser testing, and headless mode. Among all of them, Cypress follows a developer-centric approach. It has built-in features like robust DOM manipulation, headless testing capabilities, and an integrated test runner. At the same time, it provides testing automation companies with extensive language support and cross-browser testing capabilities. However, the Playwright combines the strengths of both Cypress and Selenium.
Finally, your decision should be based on your project’s unique requirements and your team’s knowledge.
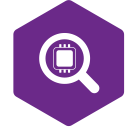
AutomationQA
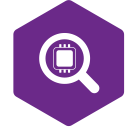