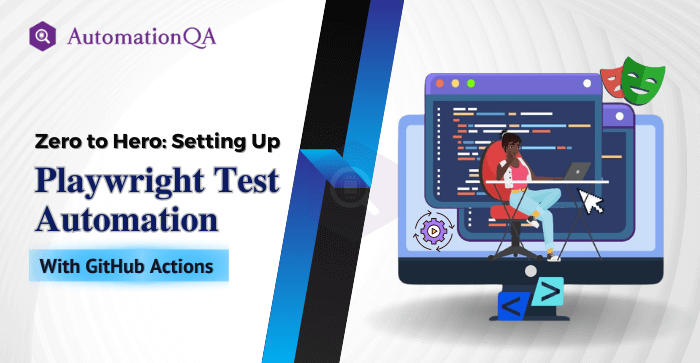
Do you know that there are around 1.13 billion websites in the world? According to Ahref, only 200 million websites are active. The remaining 90 percent of the pages get no organic traffic from search engines. But why? There can be various reasons, such as the page needing more backlinks or not targeting a topic with enough search potential. Surprisingly, however, 42 percent of people abandon a website due to its poor performance. To avoid this trap, thoroughly testing your website using the Playwright Test is crucial.
This cross-browser framework can test complex functionality across multiple languages. It can be used for parallel testing and web scraping. Playwright with GitHub Actions can automate testing, which can help in bug detection and faster feedback. Join us as we walk you through the challenges associated with website testing to achieve enhanced code quality.
Automate Like a Pro With Playwright
Playwright is a software testing tool made explicitly for testing web applications. Developers can use this tool to write test scripts in languages such as Java and C#. It supports different browser engines such as Chromium, Firefox, and WebKit, allowing scripts to run seamlessly across various browsers. This tool’s ability to test web pages’ reliability, performance, and functionality makes it unique.
Mastering Playwright GitHub Actions for Web Testing
Playwright GitHub Actions integrates the capabilities of GitHub Actions, an automation platform provided by GitHub, into the testing processes. It simplifies software development workflows by automating various tasks for developers and QA engineers. These workflows automate essential tasks like building applications, executing tests, and deploying code. Thus, developers can automate several software development life cycle processes using GitHub Actions. This way, GitHud Actions simplifies and streamlines the tasks related to software development.
Set Up CI/CD GitHub and Run Playwright Test
Following these steps, you can effectively set up a Playwright test using GitHub Actions for continuous integration and automated testing.
Step 1: Setting Up The Repository
Start by creating a new repository named “playwright_github_action” on GitHub. Initially, there needs to be code in this repository.
Example 1: Testing Web Application
Copy and paste the provided web application testing code into your preferred editor.
import { test, expect } from '@playwright/test';
test('GitHub', async ({ page }) => {
await page.goto('https://github.com/');
await expect(page).toHaveTitle('GitHub: Where the world builds software · GitHub');
// Add more test steps as needed
// Example: Check if the Sign up button is visible
await expect(page.locator('text=Sign up')).toBeVisible();
// Example: Navigate to Pricing page and verify its title
await page.click('text=Pricing');
await expect(page).toHaveURL('https://github.com/pricing');
await expect(page).toHaveTitle('Pricing · Plans for every developer · GitHub');
// Example: Search for a repository
await page.fill('input[name="q"]', 'playwright');
await page.press('input[name="q"]', 'Enter');
await expect(page).toHaveURL(/.*search.*/);
await expect(page.locator('text=playwright')).toBeVisible();
});
Example 2: API Testing
Similarly, copy and paste the provided code for API testing into your playwright testing tool code editor.
import { test, expect } from "@playwright/test";
// Assuming this global variable is to track user ID obtained from a POST request
var userId;
// Example of a GET request test
test('GET Request', async ({ request }) => {
const response = await request.get('https://your.api/endpoint');
expect(response.status()).toBe(200);
const responseBody = await response.json();
// Example assertion, adjust according to your API response structure
expect(responseBody).toHaveProperty('data');
});
// Example of a POST request test
test('POST Request', async ({ request }) => {
const response = await request.post('https://your.api/endpoint', {
data: {
// Your POST request payload here
name: 'John Doe',
email: '[email protected]'
}
});
Step 2: Commit And Push
The next step after writing the test script is to save your changes. The command “git commit -m “New tests” can accomplish this. Before running the script, share your code with others by pushing it to a repository.
Step 3: Run Tests Using GitHub Actions
Access GitHub Actions: Go to the “Actions” tab in your repository on GitHub, where you’ll see the tests you’ve committed.
Initiate Tests: To initiate the test in the playwright automation testing framework, click on the “test” box.
Step 4: Check Test Results
After running the tests, return to the “Actions” tab. If a test fails, you’ll see an error message. If it passes, a green checkmark will be displayed.
Step 5: Access Test Reports
Download Test Report: Navigate to the “New tests” section and click the “playwright-report” button at the bottom of the page to download the test report.
View Report: Extract the downloaded report file and open it in a web browser to review detailed test results and any potential issues encountered during testing.
Execute The Test Cases By Integrating GitHub Actions With Playwright Test
GitHub Actions is highly effective in automated testing due to its capabilities for custom workflows and integration with testing frameworks.
Continuous Integration (CI)
Playwright testing companies can leverage GitHub Actions to establish automated tests that run whenever a new code commit or pull request occurs. It ensures that changes keep the existing functionality of the website.
Diverse Test Environments
GitHub Actions supports running workflows on various operating systems and environments. This feature allows the QA tester to test the website across different platforms and configurations. It is crucial to ensure the website works well across all the platforms. Further, it also helps the developer to identify platform-specific bugs.
Parallel Test Execution
GitHub Actions enables running tests in parallel, reducing the time needed for test execution. This feature is crucial for large test suites, providing faster feedback.
Custom Workflows
You can create workflows tailored to your QA automation testing requirements. For instance, you can design workflows to run specific tests based on modified files in a pull request, enabling targeted testing and reducing overall testing time.
Integration With Testing Frameworks
GitHub Actions integrates with popular testing frameworks like Cypress, Appium, Selenium, etc. Thus, you can configure GitHub Actions to execute tests using your preferred testing tools.
Optimization Of Playwright Test In GitHub Actions
By implementing these optimizations, you can improve the efficiency and effectiveness of Playwright testing in GitHub Actions. This results in faster feedback on code changes and smoother testing experiences for developers and QA engineers.
Caching Dependencies
Use caching to store and reuse dependencies, like node_modules, to avoid redundant installations and speed up workflow execution.
- Example configuration using `actions/cache`:
name: Example Node.js CI
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Cache Node.js modules
uses: actions/cache@v3
with:
path: ~/.npm
key: ${{ runner.os }}-node-${{ hashFiles('**/package-lock.json') }}
restore-keys: |
${{ runner.os }}-node-
- name: Install Dependencies
run: npm ci
- name: Build
run: npm run build
- name: Test
run: npm test
Adjust Upload Artifact Condition
– Customize artifact uploads based on test outcomes to manage storage and avoid unnecessary uploads.
– Example configuration uploading artifacts only on failure:
name: Example Workflow
on: [push, pull_request]
jobs:
build-and-test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Run tests
run: |
# Replace this with commands to run your tests
# For example, for a Node.js project: npm test
echo "Running tests..."
# Add more steps as needed for your build and test process
- name: Upload Failure Artifact
if: failure()
uses: actions/upload-artifact@v3
with:
name: playwright-report
path: playwright-report/
retention-days: 30
Parallel Testing
Reduce the amount of time spent testing overall by dividing the test suite into several smaller jobs that can run concurrently on different runners. Make use of GitHub Actions’ matrix approach to run concurrent tasks in various configurations. Example matrix configuration running tests in parallel across different operating systems:
name: Cross-OS Tests
on: [push, pull_request]
jobs:
test:
runs-on: ${{ matrix.os }}
strategy:
matrix:
os: [ubuntu-latest, macos-latest, windows-latest]
steps:
- name: Checkout repository
uses: actions/checkout@v2
- name: Set up Python # Example setup step, adjust according to your project needs
uses: actions/setup-python@v2
with:
python-version: '3.8' # Specify the Python version you need
- name: Install dependencies
run: |
pip install -r requirements.txt # Adjust this command based on your project's dependency management
- name: Run tests
run: |
# Replace the following command with your test command
pytest tests/
Publish Reports to GitHub Pages
Publish test findings so your team members can easily access and examine the results. To deploy the report, enable GitHub Pages and set up GitHub Actions using `actions/configure-pages` and `actions/deploy-pages`.
– Example configuration for deploying to GitHub Pages:
name: Deploy Report to GitHub Pages
on:
push:
branches:
- main # Adjust this to match the branch you want to trigger the deployment from
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Checkout repository
uses: actions/checkout@v2
- name: Setup Pages
uses: actions/configure-pages@v3
- name: Upload artifact
uses: actions/upload-pages-artifact@v2
with:
path: "playwright-report/"
- name: Deploy to GitHub Pages
uses: actions/deploy-pages@v2
Final Words
Combining the Playwright test with GitHub Actions can streamline the web development process. You can seamlessly conduct end-to-end testing with Playwright’s robust browser automation features and GitHub Actions’ adaptable CI/CD automation. Additionally, it ensures cross-browser compatibility and identifies issues at an early stage of development. Thus, you can optimize the testing and deployment pipeline by integrating Playwright with GitHub Actions.
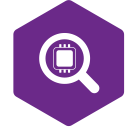
AutomationQA
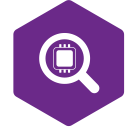