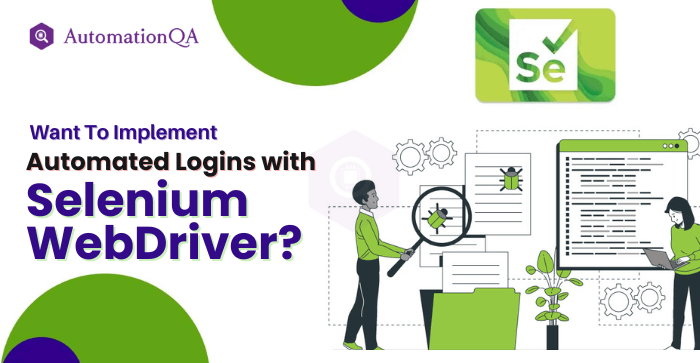
Selenium is the first tool that comes to mind when discussing automation testing. Selenium WebDriver allows users to write their tests in their preferred programming language, so it is used to automate website tests. Login is one of the most essential things that every website may offer. Although the login feature appears less critical, it is crucial for the users’ privacy. Because it is the gateway to the user’s sensitive information, a designer must ensure its reliability through comprehensive testing. It would be hard to believe, but any disruption in the login function can result in a loss in revenue.
In this blog article, we will examine how to use Selenium to automate the testing of this feature. Through Selenium test automation, let’s ensure a smooth login for your users.
Requirement Of Automating Login Feature Using Selenium WebDriver
You’ll need the following prerequisites to automate the testing of the login feature:
1. Download And Install JDK (Java Development Kit)
Go to the Oracle website to download and install the JDK version that is compatible with your operating system.
Configure the JDK installation directory as the pointer for the JAVA_HOME environment variable.
2. Download And Install IDE (Integrated Development Environment)
– Installing an IDE like Eclipse or IntelliJ IDEA from their official website to write and execute your Selenium test scripts.
3. Integrate TestNG With IDE
– TestNG is a testing framework that provides additional functionalities to testing tools.
– Install the TestNG plugin in your IDE or manually add TestNG to your project’s dependencies.
4. Selenium Client Configuration
– Download the Selenium WebDriver client libraries from the Selenium website.
– Configure the Selenium client libraries in your project’s build path in your IDE.
5. Browser Drivers
– Download browser-specific WebDriver executables (e.g., ChromeDriver, GeckoDriver for Firefox, EdgeDriver) from their official websites.
– Place them in a directory in your system’s PATH environment variable or specify the WebDriver executable path in your Selenium script.
Once these prerequisites are set up, you can start writing Selenium WebDriver scripts to automate the login process. You can use Java programming language and WebDriver APIs to interact with web elements to perform actions like entering credentials, clicking buttons, and verifying login success.
Steps For Automate Login Functionality
Step 1- Incorporate The WebDriver
You must instantiate the WebDriver object for the specific browser you intend to automate. Here’s how you can do it for Chrome, Firefox, and Edge browsers:
For Chrome:
```java
System.setProperty("webdriver.chrome.driver", "path_of_chromedriver");
WebDriver driver = new ChromeDriver();
```
For Firefox:
```java
System.setProperty("webdriver.gecko.driver", "path_of_geckodriver");
WebDriver driver = new FirefoxDriver();
```
For Edge:
```java
System.setProperty("webdriver.edge.driver", "path_of_edgedriver");
WebDriver driver = new EdgeDriver();
```
Selenium automation companies can replace `”path_of_chromedriver”`, `”path_of_geckodriver”`, and `”path_of_edgedriver”` with the actual paths where their ChromeDriver, GeckoDriver (for Firefox), and EdgeDriver are located on their system. This code sets the system property for the respective driver. Then, it instantiates the WebDriver object for the specified browser, which allows companies to automate tasks with the chosen browser.
Step 2- Maximization Of Browser
To maximize the browser window in Selenium, you use the `manage().window().maximize()` method. It ensures that test scripts can execute without encountering any errors. Here is an example of how Selenium companies use it to maximize the browser window:
```java
driver.manage().window().maximize();
```
After executing this line of code, the browser window will be maximized, providing an optimal view for test execution and interaction with web elements.
Step 3- Navigation Through URL
To navigate to a URL after opening the browser in maximize mode, you can use the `get()` method. This method typically takes a string parameter, the URL of the tested application, and returns void.
Here’s an illustration of how to use Selenium’s `get()` method to navigate to a URL:
```java
driver.get("URL of the application/Website");
```
After executing this line of code in the Selenium automation testing, the browser will navigate to the specified URL, allowing the test script to interact with the desired web application.
Step 4- Synchronism Using Implicit Wait
Synchronization is crucial in automation testing to handle situations where applications may take longer to load, potentially causing script failures. Selenium addresses this by introducing implicit waiting. It allows testers to set a maximum time to wait for an element to appear. In the following example, let’s see how you can add a maximum wait of 30 seconds for each component:
```java
driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS);
```
This code will ensure that Selenium waits for the specified time before proceeding with the script. It helps the Selenium automation testing company to prevent failures due to element unavailability during dynamic loading.
Step 5- Locating Web Elements Using Selenium
It involves employing various techniques based on the attributes and structure of the HTML elements on a webpage. These are the main techniques:
1. ID: A unique element identifier.
2. ClassName: Class attribute of an element.
3. Name: Name attribute of an element.
4. XPath: Expression to locate elements in XML documents.
5. TagName: The HTML tag name for an element.
6. CssSelector: CSS path to an element.
7. LinkText: A link’s exact text.
8. PartialLinkText: A link’s partial text.
Selenium WebDriver can locate elements effectively for automation tasks based on the specific attribute or structure.
Step: 6- Verification And Validation
These are crucial steps in testing as they compare the actual outcomes with the expected ones. If they do, the test case passes; otherwise, it fails.
Selenium Test Case For Automating The Login Process
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.Assert;
import org.testng.annotations.Test;
public class Sample {
@Test
public void TestSigmaLogin() {
// Set up Chrome driver
System.setProperty("webdriver.chrome.driver", "path of driver");
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
// Open Sigma login page
driver.get("https://app.testsigma.com/ui");
driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS);
// Enter login credentials
driver.findElement(By.id("loginUsername")).sendKeys("[email protected]");
driver.findElement(By.name("password")).sendKeys("xxxxxxx");
driver.findElement(By.xpath("//button[text()='SIGN IN']")).click();
// Verify the URL after login
String actualURL = "https://xyz.com/home";
String expectedURL = driver.getCurrentUrl();
Assert.assertEquals(expectedURL, actualURL);
// Close the browser
driver.close();
}
Final Words
Login functionality is critical to any website, and testing it is straightforward with Selenium WebDriver. Automation allows for comprehensive testing, efficiently covering boundary values, edge cases, and negative and positive scenarios. This article demonstrates how to implement login functionality effectively using Selenium test automation services. We have also provided a sample code to familiarize yourself with the login testing process.
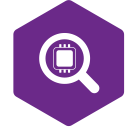
AutomationQA
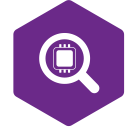